The quick method I’m utilizing is drawing a polyline along the profile in a profile view. I’ve hard coded the values I need, but also added the code to prompt the user for the information.
1: Sub PerformProfileDemonstration()
2:
3: ' Always get the objects again since MDI is supported.
4: If (GetBaseCivilObjects() = False) Then
5: MsgBox "Error accessing top civil objects."
6: Exit Sub
7: End If
8:
9: Dim oAcadObj As AcadObject
10: Dim vPt As Variant
11:
12: ThisDrawing.Utility.GetEntity oAcadObj, vPt, "Pick Profile: "
13:
14: Dim dAxelHt As Double
15: dAxelHt = 1.5 ' ThisDrawing.Utility.GetReal("Enter Axel Height: ")
16:
17: Dim dLength As Double
18: dLength = 35 ' ThisDrawing.Utility.GetReal("Enter Length between Axels: ")
19:
20: If (TypeOf oAcadObj Is AeccProfile) Then
21:
22: Dim oProfile As AeccProfile
23: Set oProfile = oAcadObj
24:
25: ThisDrawing.Utility.GetEntity oAcadObj, vPt, "Pick Profile View: "
26: Dim oProfileView As AeccProfileView
27: If (TypeOf oAcadObj Is AeccProfileView) Then
28: Set oProfileView = oAcadObj
29: Else
30: Exit Sub
31: End If
32:
33: Dim dStartSta As Double
34: Dim dStaInt As Double
35: Dim dEndSta As Double
36:
37: dStartSta = 250 ' ThisDrawing.Utility.GetReal("Enter starting Station: ")
38: dEndSta = 400 'ThisDrawing.Utility.GetReal("Enter Ending Station: ")
39: dStaInt = 2 ' ThisDrawing.Utility.GetReal("Enter Station interval: ")
40:
41: Dim oPoly As AcadLWPolyline
42: Dim dPoints(0 To 7) As Double
43: Dim dStartElev As Double
44: Dim dEndElev As Double
45: Do Until dStartSta > dEndSta
46:
47: dStartElev = oProfile.ElevationAt(dStartSta)
48: dEndElev = oProfile.ElevationAt(dStartSta + dLength)
49:
50: ' Get the points on the profile view, it will account for vertical exageration.
51: oProfileView.FindXYAtStationAndElevation dStartSta, dStartElev, dPoints(0), dPoints(1)
52: oProfileView.FindXYAtStationAndElevation dStartSta, dStartElev + dAxelHt, dPoints(2), dPoints(3)
53: oProfileView.FindXYAtStationAndElevation dStartSta + dLength, dEndElev + dAxelHt, dPoints(4), dPoints(5)
54: oProfileView.FindXYAtStationAndElevation dStartSta + dLength, dEndElev, dPoints(6), dPoints(7)
55:
56: ThisDrawing.ModelSpace.AddLightWeightPolyline dPoints
57:
58: dStartSta = dStartSta + dStaInt
59: Loop
60:
61: End If
62:
63: End Sub
The output looks something like this:
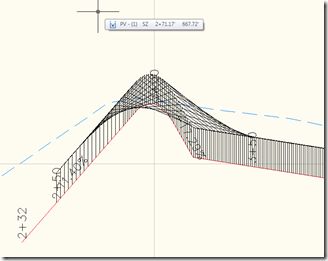
As you can see my vertical curve is too steep for the vehicle. You’ll also notice that I haven’t written the code for the tires to be perpendicular to the profile. In order to get realistic values I probably should revise the code to account for this. Since I don’t have that much time available to day I might leave that for another post or maybe you can come up with a way to do it. The hard part isn’t getting the first tire to be perpendicular, but finding out where the other other tire should sit and be perpendicular to the surface. Fortunately the difference isn’t that much so this method should give a close enough answer. In the picture above the difference was 0.097’.
No comments:
Post a Comment